Technical Specifications
System Diagram
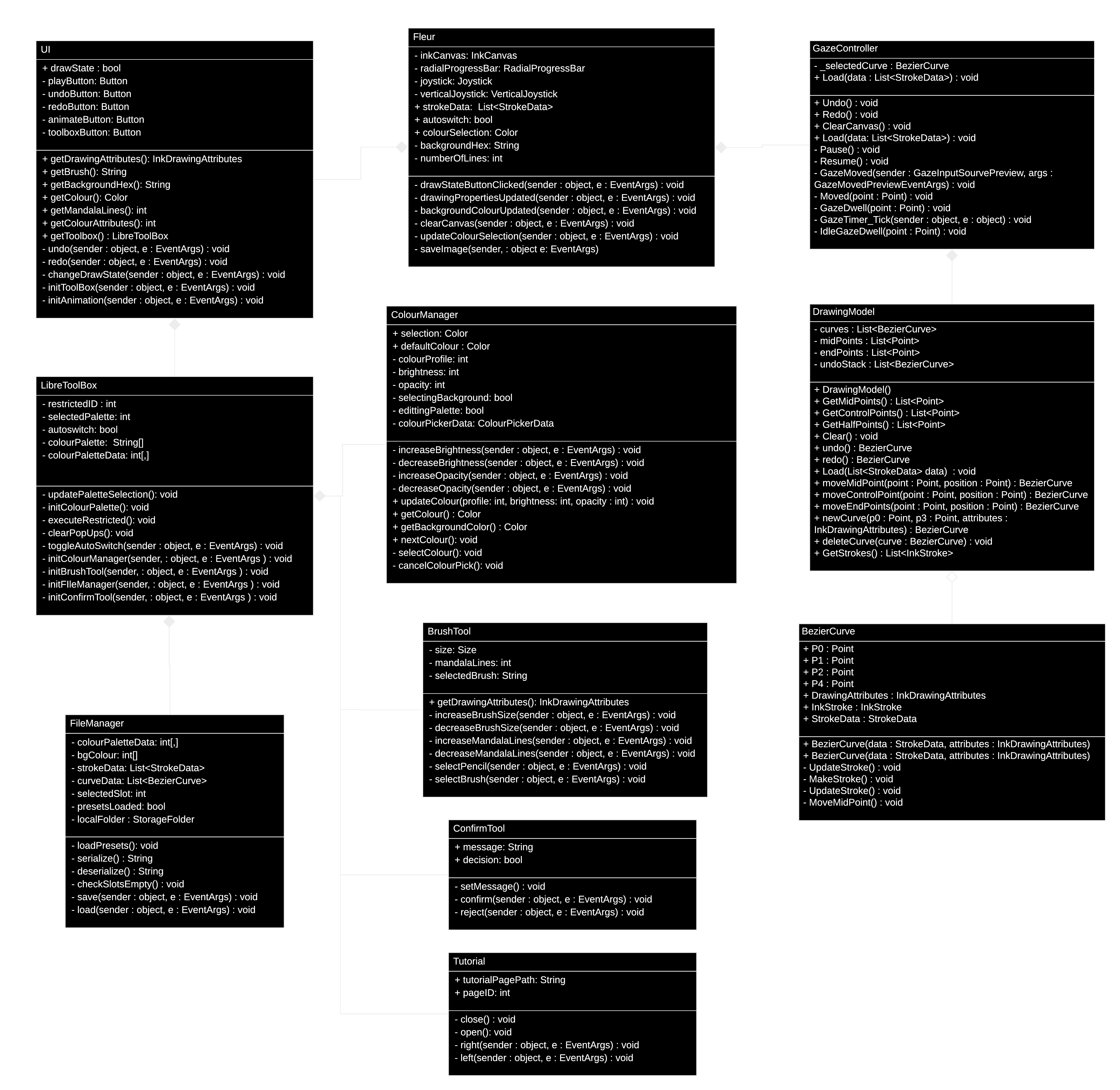
Gaze/Canvas Interaction Mechanism
The Gaze Interaction Library provided by the Windows Community Toolkit does not support dwelling for arbitrary points on a canvas. We implemented several functions to support this essential functionality.
If the distance between the gaze point is less than 5 pixels, the timer will be started. The timer value will accumulate until GazeDwell is called or the gaze point is moved to other location.
int TimerValue = 0
Point GazePoint = EyeTracker.GetGazePoint()
func GazeMoved(Point point):
dx = point.X – GazePoint.X
dy = point.Y – GazePoint.Y
distance = sqrt(dx * dx + dy * dy)
if(distance < 5 and !TimerStarted):
TimerValue = 0
Timer.Start()
TimerStarted = true
if(distance >= 5 and TimerStarted):
Timer.Stop()
TimerStarted = false
func GazeTimer_Tick():
TimerValue += 20
if(TimerValue >= 600):
GazeDwell()
Testing
Avant-Garde’s system architecture is divided into two sections, the Drawing Mechanism and the UI. The first contains the code which drives the interaction between the eye-tracking device input and the output unto the ink canvas. The majority of the development of this section involved programming functionalities unto several joysticks, utilising Bezier Curves to create curved lines, and interpreting the Eye-Tracker’s output. Seeing as these functionalities depend on Eye-Tracking input to be tested, user testing was utilised to ensure the expected program behaviour was achieved in all cases.
The UI section of the application contains the front end for all menus, the buttons that trigger a large proportion of the application’s functionalities, and it propagates data (colours, brush attributes, etc.) from individual User Controls (such as the Colour Manager), to the Fleur class, which has access to the Drawing Mechanism. As the front end of the app was developed in XAML using Visual Studio, all its designs could be tested real time as they were developed. As for the backend, the majority of it involved interactions between multiple classes in parent-child relationships, which again naturally lent itself to user system testing.
Mandala Generation Algorithm
In UWP, strokes on the InkCanvas are instances of class InkStroke which consist of numerous InkPoint objects. The function TransformPoint transforms a single InkPoint into a list of InkPoints which are the results of rotating the point around the center point of the window, generating mandala designs from a single stroke. As the name suggests, TransformStroke transforms a single InkStroke into a list of InkStrokes which are the results of rotating the stroke.
func TransformPoint(point, number):
transformed_points := empty list
angle := 2 * pi / number
point_polar := polar form of point
for i = 0 to number:
new_point_polar := new polar object
new_point_polar.angle := point_polar.angle + i * angle
new_point_polar.magnitude := point_polar.magnitude
add new_point_polar into transformed_points
return transformed_points
func TransformStroke(stroke, number):
transformed_strokes := empty list
for i = 0 to number:
for point in stroke:
transformed_points := TransformPoint(point, number)
new_stroke := BuildStroke(transformed_points)
add new_stroke into transformed_strokes
return transformed_stroke
Additional Specifications
Integrated APIs
Name | Link |
---|---|
Colour Picker: | https://docs.microsoft.com/en-us/windows/uwp/design/controls-and-patterns/color-picker |
Gaze Interaction Library: | https://docs.microsoft.com/en-us/windows/communitytoolkit/gaze |
Ink Canvas: | https://docs.microsoft.com/en-us/uwp/api/Windows.UI.Xaml.Controls.InkCanvas |
Ink Toolbar: | https://docs.microsoft.com/en-us/uwp/api/windows.ui.xaml.controls.inktoolbar |
Bezier Curve: | https://docs.microsoft.com/en-us/uwp/api/windows.ui.xaml.media.beziersegment |
Supported Eye-Tracking Devices
Name |
---|
Tobii Eye Tracker 4C (Used for development and Testing) |
Tobii EyeX |
Tobii Dynavox PCEye Plus |
Tobii Dynavox EyeMobile Mini |
Tobii Dynavox EyeMobile Plus |
Tobii Dynavox PCEye Mini |
Tobii Dynavox PCEye Explore |
Tobii Dynavox I-Series+ |