Implementation
Web Application Implementation
Main Technology
PYTHON AND FLASK
We used Python as our main language and Flask along with it in order to build our web application.
AZURE
We used tools for Azure to aid our text summarisation process; we used the Azure Speech SDK in order to transcribe uploaded audio to text which would then be fed into one of our 18 machine learning algorithms. Also, we use Azure Virtual Machine to be the host of our SQL server.
SQL SERVER
SQL Server was used in order to create our relational database, and store and fetch data through SQL queries. And we install the SQL Server on the 20.04 Ubuntu which connects to the Azure Virtual machine.
DATABASE
Our first task was planning our relational database, and creating a suitable database design to suit our client’s needs. Based on our client’s requirements, we planned the following tables:
- User
- Role
- File
*Click on the buttons inside the tabbed menu
Entity Relationship Diagram:
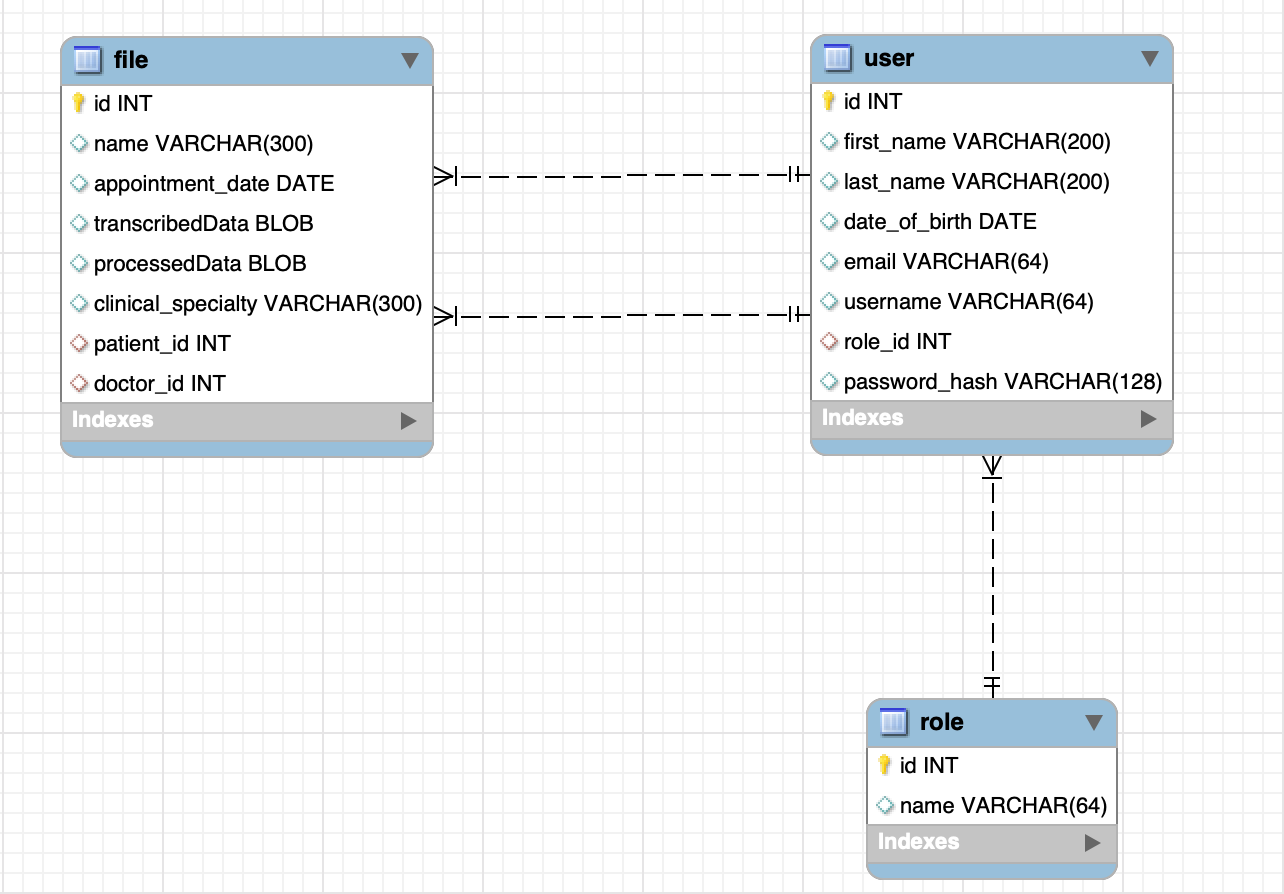
These databases were created through SQL-Alchemy in flask within a “models.py” file. All data related operations would be carried out within this layer.
Database Development
We had initially used ‘Azure Databases for MySQL’ to deploy our database however the monthly cost of this database was realised too expensive for our client. Hence, we deployed the database on a Linux Virtual Machine on Azure. This was much cheaper, and we were able to connect to the database server through its public IP address and port.
Site Map:
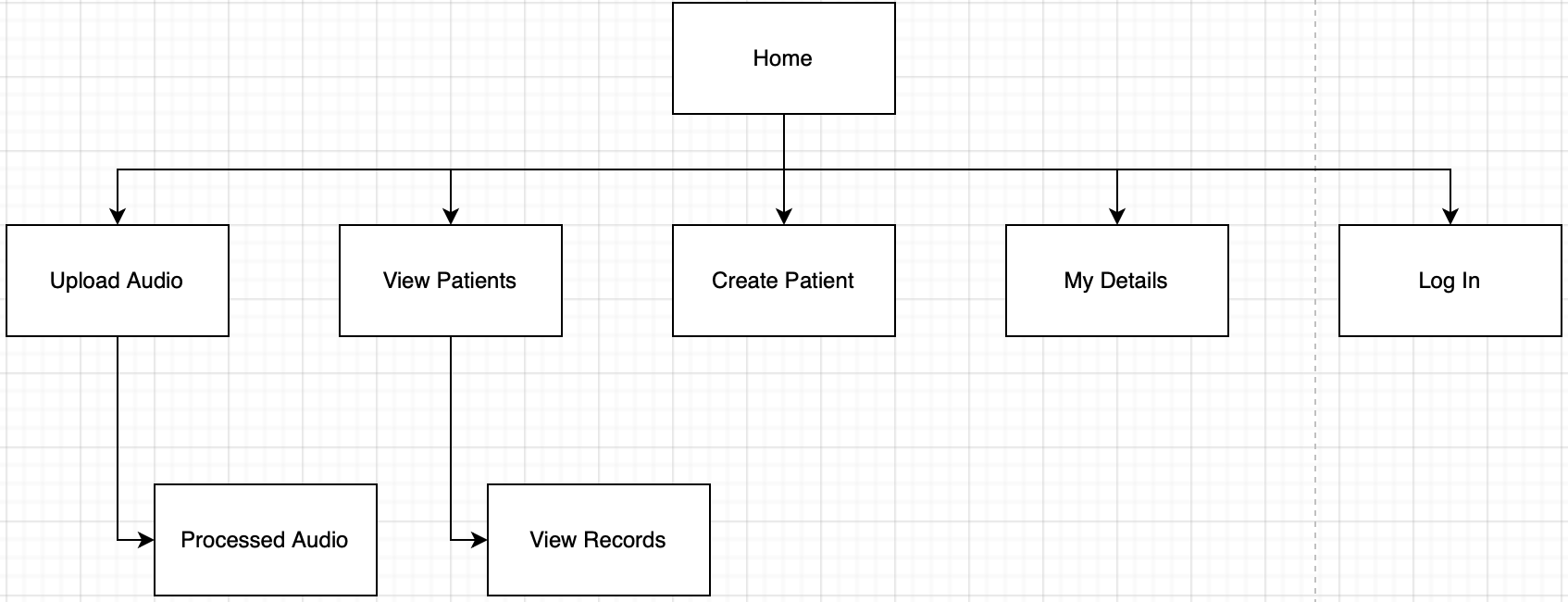
The diagram above is a site map showing all the main pages in our application.
System Architecture Diagram:
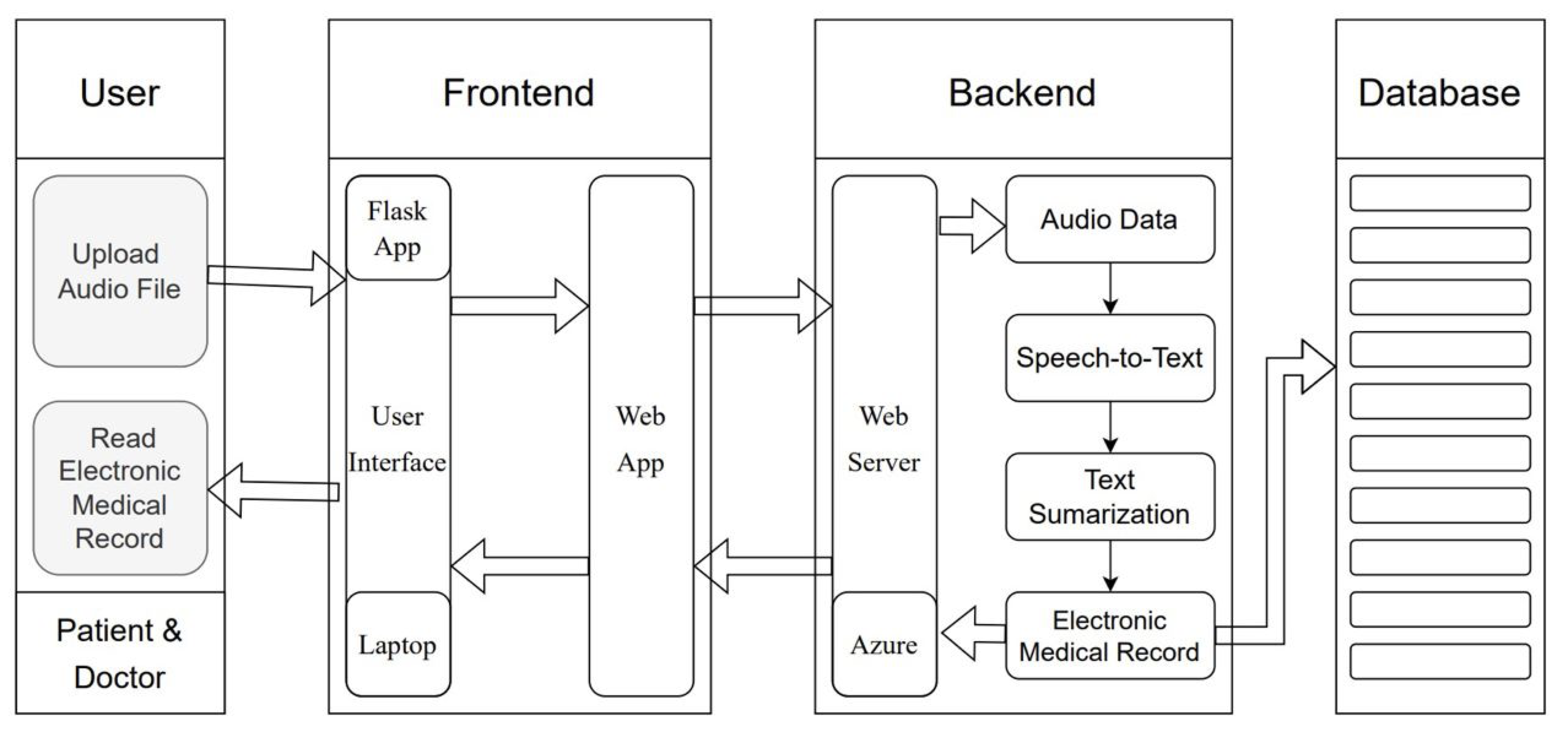
The diagram above is a site map showing all the main pages in our application.
Uploading Audio:
The core functionality of our application was to accurately summarise transcribed audio in order to general a summarised clinical report (the Electronic Medical Record).
When the doctor uploads audio, along with:
- Entering the date of the appointment that the consultation audio came from
- Clinical Specialty
- Patient (selecting the patient speaking in the consultation audio)
Once this is all submitted on the web form, the following process occurs:
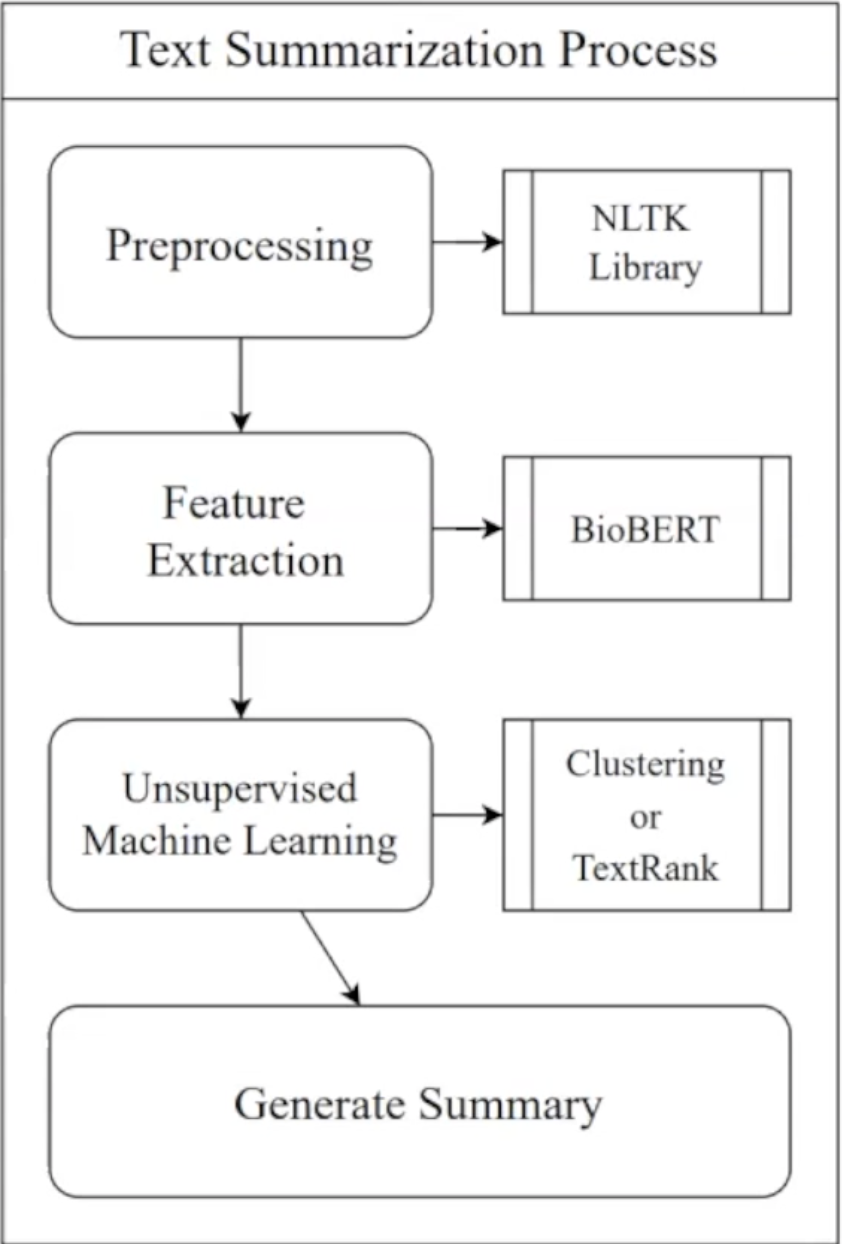
During this process, 18 machine learning algorithms are selected and evaluated in the system.
Once the summary is generated, the doctor can confirm whether they are satisfied with the transcribed audio and the summary.
If the doctor confirms their satisfaction, then the transcribed audio and the summary are stored in the File table of the database with the ID of the patient speaking in the consultation audio (this ID links to the User table), the ID of the doctor (this ID links to the User table too), the appointment’s date, and the clinical speciality. If the doctor is not satisfied, then it is not stored in the database and the doctor is taken back to the home page.
Security and Authentication:
Maintaining patient-confidentiality was a big focus of our application, due to the legal implications that could be brought from its breach.
Hence, we ensured the following:
- All patient records can only be accessed by doctors
- Doctors can only upload audio (which is then transcribed and summarised) to process and store in the database as records
- Patient users are only created by doctors
- Passwords for users are hashed when storing in the database
The first three objectives were achieved by implementing role-based authorisation. If a user was found to not have the required role for accessing a particular url (which then renders a HTML page), then they would be denied access. This was implemented by creating a decorator called requires_roles which I could place above any function that renders a html page; restricting access to users with a particular role.
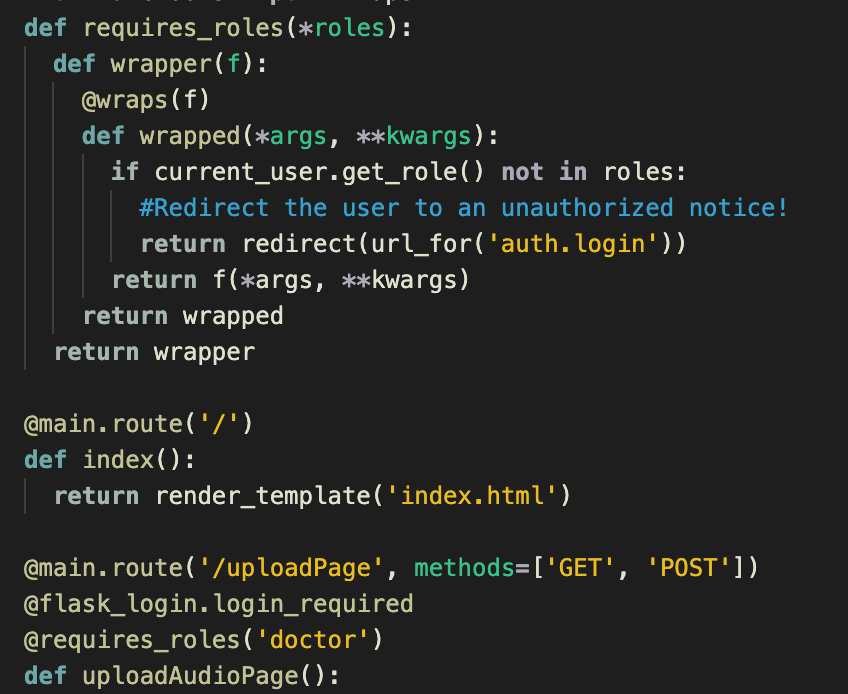
Passwords for users are also hashed when stored in the database.
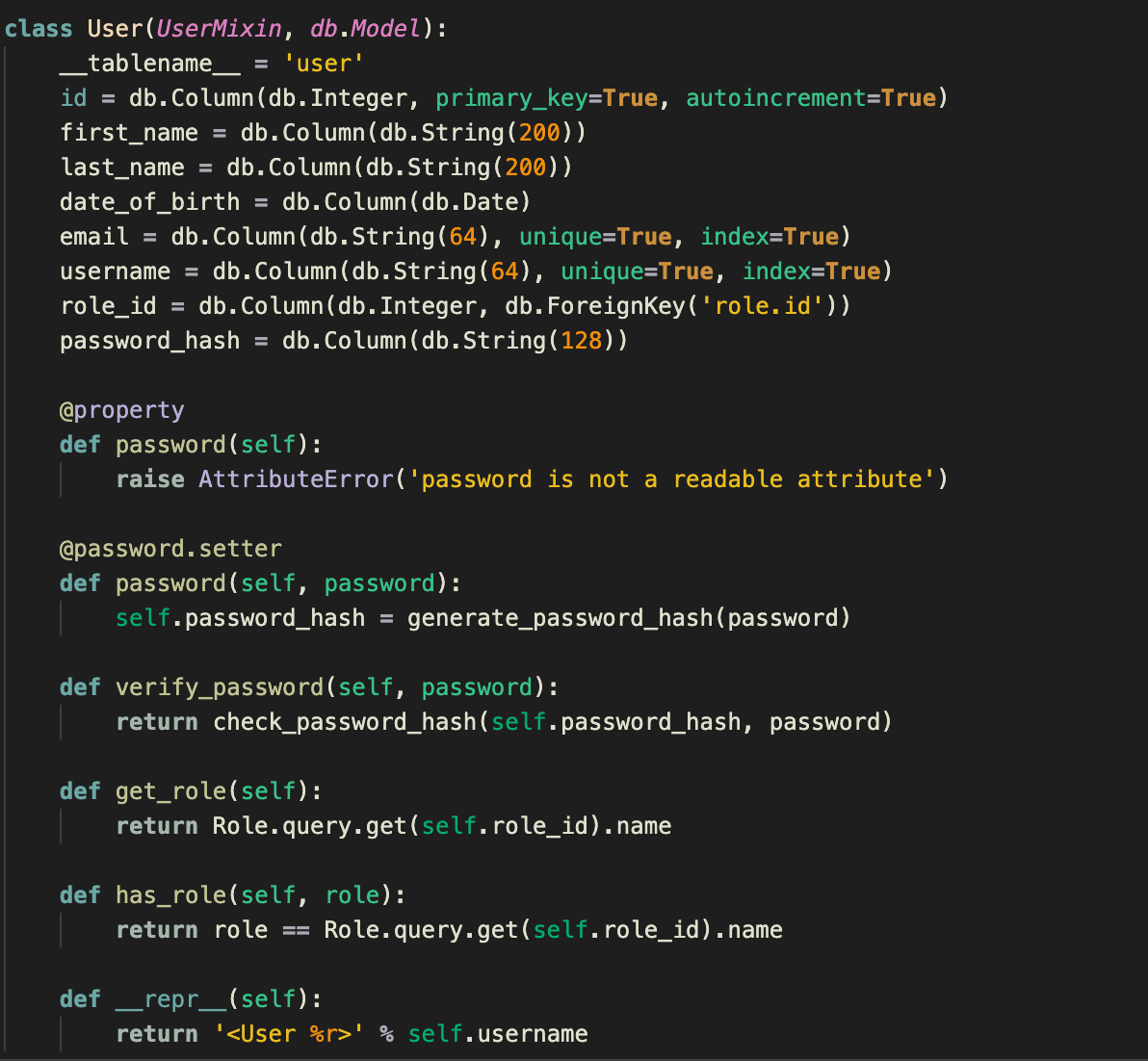
The code above ensured that when a User object would be created, then the string within the attribute containing the password would be hashed in the password method which contains the @password.setter decorator. Hence when a new user is added and committed to the User table in the database, then the hashed password would be stored in the database.
This was particularly important, as hashing passwords provides defence against users’ passwords being compromised when a database has been compromised.
Class Diagrams:
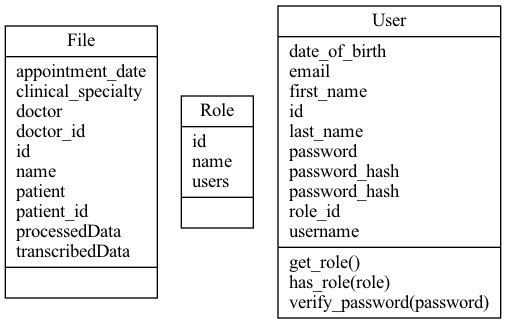
The classes above are made to map to their respective tables in the database; this is used with SQL-Alchemy which is an SQL toolkit and object relational mapper.
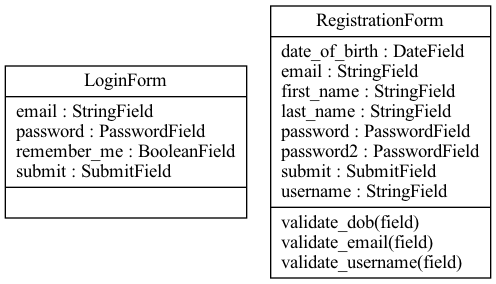
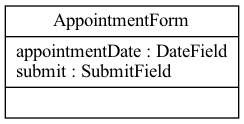
The classes above were used for loading their respective forms on html pages rendered to the user. Although many forms were created on the client-side, sometimes it was easier to create the form on the server-side as classes and also code for validation on the server-side.

The classes above were used for configuring our application, as it starts up.