Class Diagram
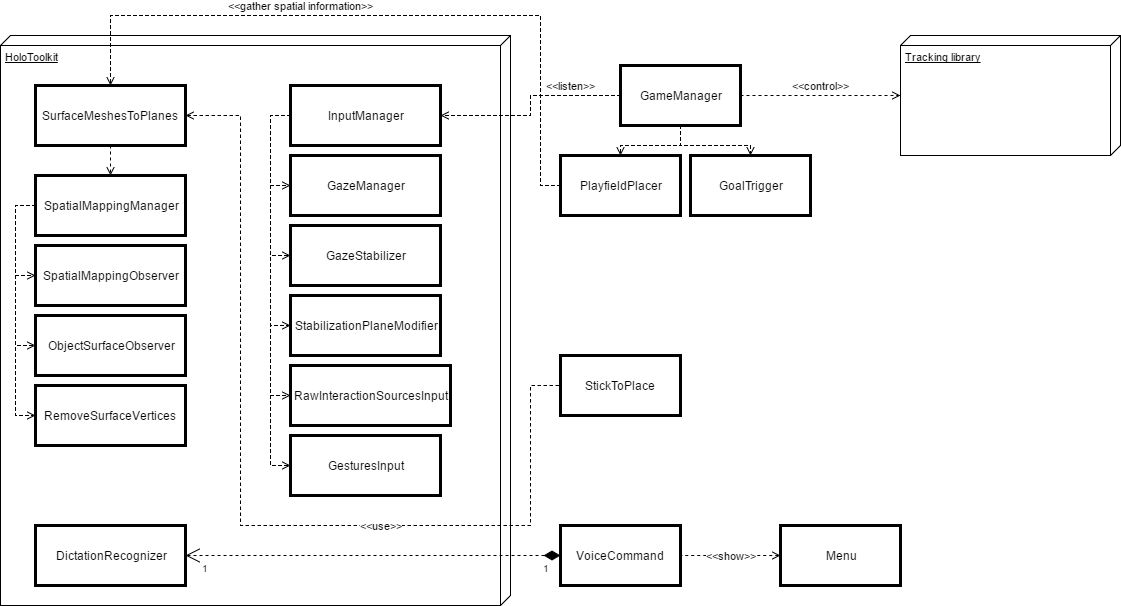
Design Pattern
We are utilising several common design patterns in our application – some borrowed from the frameworks we are using, some stemming from our experience. They help other developers recognize our code and us to abstract information away into more extensible concepts as opposed to reinventing the wheel with suboptimal design choices.
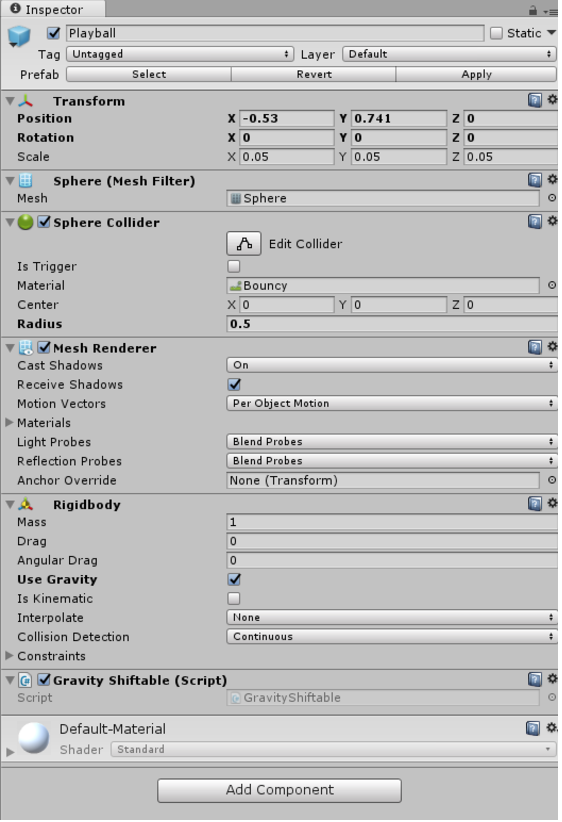
Singleton pattern
HoloToolkit-Unity (by Microsoft), the library we are using for developing in Unity for the HoloLens, uses singletons extensively. As such, we also follow their ideology with using their singletons. The singleton pattern is useful for avoiding supplying a long list of references to each object that needs a big manager class. That class can be made a singleton (if it makes sense to do so – i.e. it must be singular and perpetual) and the reference can be accessed statically.
Observer pattern
This is a very common pattern, that we have also employed. Mainly used for event/action listeners, and our project was no different. The HoloToolkit input manager design uses custom interfaces for classes that want to listen to input events from the HoloLens, for example “tap” events, or “manipulation” gestures. This uses a system of global and modular listeners, which objects can use to subscribe to the input manager singleton instance to receive events accordingly to their needs. Also, our game uses events like onGameReset, onPlayfieldPlaced and so on, that objects can subscribe to and dictate their behaviour.
Factory pattern
We have used the weaker version of the factory method pattern, where we utilized functions for automatically generating instances depending on the input arguments to the factory function. This is useful for abstracting away implementation details of how the instances are set up. That in turn helps to maintain a cleaner codebase.
Composite pattern
Unity uses the composite pattern for all its GameObjects. These can contain components, which can provide rendering, collision, animation, or scripting functionality (among other things). This helps to treat collections of objects as a single entity. The advantages include modular behaviour for the objects and a tree hierarchy for the level, where increasingly complex structures can be slowly built from simpler ones.
Prototype
Another design patter borrowed from Unity’s design is that of a prototype – called a prefab in Unity. This allows a prototypical theoretical instance of a GameObject to exist, which can then be copied and it has all (or most) of the required functionality set up. This is supported by the Instantiate function in Unity, which takes as an argument an instance of a GameObject and then copies it with all its components. We use this for example for creating our UI buttons, setting up the basic level with all the prerequisites, for highlighting places suitable for playing our game (like tables), and for quickly designing our levels in the Unity editor.